Importing an SVG file as a React component and importing it as a regular image file
August 11, 2023 7:53 PM
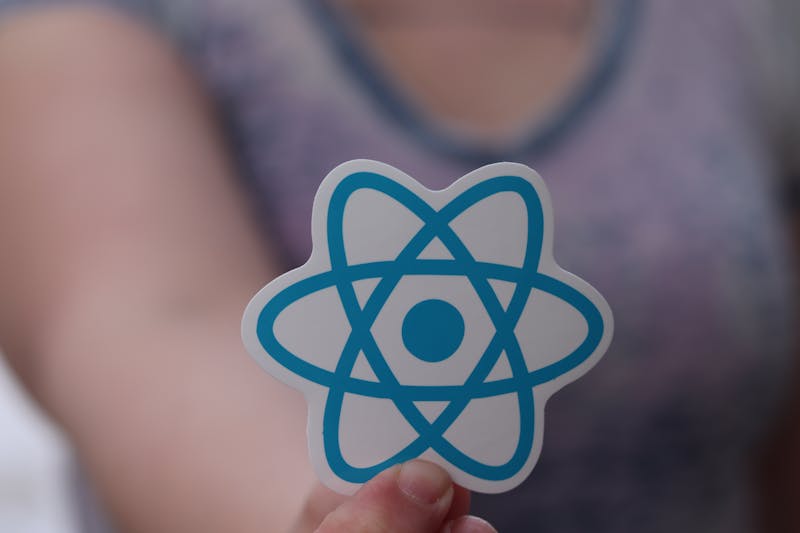
When importing an SVG file as a React component, the SVG file is treated as a component that can be manipulated with props and state. This allows for more flexibility and control over the SVG, as it can be customized and animated using React's built-in features.
On the other hand, when importing an SVG file as a regular image file, the SVG is treated as a static image that cannot be manipulated with props and state. This limits the flexibility and control over the SVG, as it cannot be customized or animated using React's built-in features.
Additionally, importing an SVG file as a React component allows for better performance and accessibility, as the SVG can be rendered as an inline SVG rather than an external file. This reduces the number of HTTP requests and improves the accessibility of the SVG for screen readers and other assistive technologies.
Overall, importing an SVG file as a React component is a more powerful and flexible approach, while importing it as a regular image file is a simpler and more limited approach.
Here's an example of importing an SVG file as a React component:
import { ReactComponent as HeartIcon } from './heart.svg';function App() {return (<div><h1>My App</h1><HeartIcon /></div>);}
In the above example, the heart.svg
file is imported as a React component using the ReactComponent
syntax. The HeartIcon
component can then be used in the JSX code like any other React component.
Here's an example of importing an SVG file as a regular image file:
import heartImage from './heart.svg';function App() {return (<div><h1>My App</h1><img src={heartImage} alt="Heart" /></div>);}
In this above example, the heart.svg
file is imported as a regular image file using the import
syntax. The heartImage
the variable can then be used as the src
attribute of an img
element in the JSX code.
Disadvantage: You can not customize it as per your need.
Using React with Vite:
Using the import
statement to import SVG files in a Vite app is that Vite does not support importing SVG files as modules by default.
To fix this issue, We can have different approaches .
Option: 1
- You can use a Vite plugin like
vite-plugin-svg-icons
orvite-plugin-react-svg
to enable importing SVG files as React components. These plugins will convert the SVG files into React components that can be used in your code like any other React component.
Here's an example of how to use the vite-plugin-react-svg
plugin to import an SVG file as a React component:
Install the
vite-plugin-react-svg
plugin:npm install vite-plugin-react-svg --save-dev
2. Add the plugin to your Vite config file (vite.config.js
):
import reactSvg from 'vite-plugin-react-svg';
export default {
plugins: [
reactSvg()
]
}
With this setup, the vite-plugin-react-svg
the plugin will convert the heart.svg
file into a React component
Option 2:
You can use another vite plugin like vite-plugin-svgr that uses svgr under the hood to transform SVGs into React components.
Simply install it using npm or yarn.
npm i vite-plugin-svgr
Then go to the file vite.config.js and simply do this .
Now this code will work.
import { ReactComponent as HeartIcon } from "./assets/heart.svg";
Using CRA (Create React App):
Importing SVG files as regular image files using the import
statement should work with Create React App (CRA) without any additional configuration.
CRA uses Webpack under the hood, which has built-in support for importing SVG files as image files. When you import an SVG file using the import
statement, Webpack will automatically handle the file and include it in the build output.
Webpack, for instance, has a loader for handling SVGs called file-loader. So, our code is still valid in the CRA project without any issues.
https://codesandbox.io/s/funny-hermann-wwggpf (Codesandbox demo)
Comments